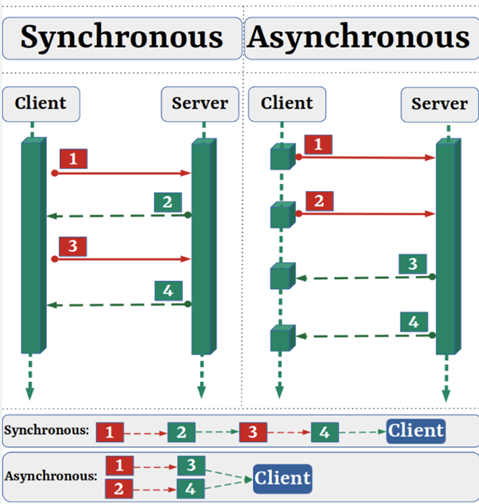
Mastering Asynchronous Programming in Python: A Comprehensive Guide
Asynchronous programming in Python is an essential tool for developers who want to create highly efficient and scalable applications. With the ability to handle multiple tasks at once, asynchronous programming allows your program to perform non-blocking operations, which can significantly improve the performance of I/O-bound and high-latency operations.
In this comprehensive guide, we will dive deep into the core concepts of asynchronous programming in Python, explore the usage of async
and await
keywords, learn about event loops, and understand how to implement concurrency to make your code more efficient. This guide is aimed at experienced developers looking to leverage Python’s asyncio library to build faster and more responsive applications.
By the end of this article, you’ll be familiar with:
- The core principles of asynchronous programming.
- How
async
andawait
work. - The concept of event loops and tasks.
- How to use asyncio to handle concurrency effectively.
Let’s begin our journey into mastering asynchronous programming!
Table of Contents
- What is Asynchronous Programming?
- Why Use
asyncio
? - Understanding
async
andawait
- Event Loops and Tasks
- Handling Concurrency with
asyncio
- Best Practices for Asynchronous Programming
- Conclusion
What is Asynchronous Programming?
Asynchronous programming is a method of writing code that allows tasks to run independently from the main program flow. In contrast to synchronous programming, where each task must complete before moving to the next, asynchronous programming allows multiple tasks to progress simultaneously. This technique is especially useful in scenarios where tasks are I/O-bound or when waiting for external resources like network responses, file I/O, or user inputs.
When implemented correctly, asynchronous programming can significantly reduce waiting times and improve the overall efficiency of your applications.
“The art of writing efficient code lies in making the most out of time and resources, and asynchronous programming is the key to unlocking that potential.” – Donald Knuth
Why Use asyncio
?
Python’s asyncio
library is the cornerstone of asynchronous programming in Python. It provides the infrastructure for writing single-threaded concurrent code using coroutines, making it ideal for handling I/O-bound operations like database queries, API requests, and file handling.
asyncio
helps developers manage a multitude of tasks without the need for multi-threading, making it more memory-efficient and less error-prone than traditional concurrency models. The ability to run multiple operations in a non-blocking manner gives your applications the speed boost needed to handle many tasks simultaneously.
To install asyncio
, you don’t need to install any external libraries, as it comes built into Python 3.4 and later versions. To check your Python version, run:
python --version
If you’re running an older version of Python, consider upgrading to at least version 3.7 for optimal support. You can download the latest Python version here.
Understanding async
and await
The async
and await
keywords are the building blocks of asynchronous programming in Python. They are used to define asynchronous functions and handle coroutines. A coroutine is a function that can pause its execution and return control to the event loop, allowing other tasks to run in the meantime.
Here’s an example of how to use async
and await
:
import asyncio
async def fetch_data():
print("Start fetching data")
await asyncio.sleep(2)
print("Finished fetching data")
async def main():
await fetch_data()
asyncio.run(main())
In the above example, await
pauses the execution of fetch_data()
while it waits for asyncio.sleep(2)
to complete, allowing other tasks to run in the meantime. The asyncio.run()
function is used to execute the coroutine.
Understanding how async
and await
work is critical to mastering asynchronous programming. It allows you to write code that doesn’t block the execution of other tasks, which is crucial for building responsive and scalable applications.
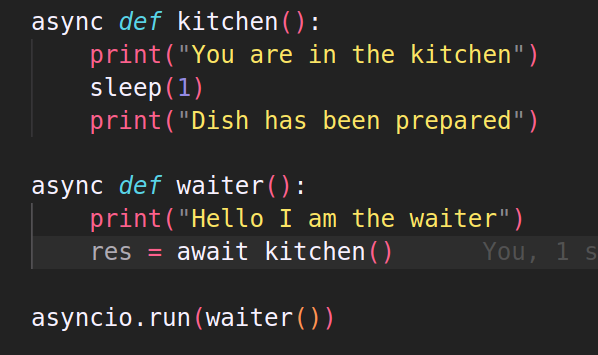
Event Loops and Tasks
The event loop is a key component of asynchronous programming. It is responsible for executing asynchronous tasks and managing their states. An event loop continuously checks for new tasks to run and handles their execution in a non-blocking manner.
In Python, the event loop is managed by asyncio
. You can create tasks using asyncio.create_task()
, which schedules a coroutine to be run in the background. Here’s an example:
import asyncio
async def task1():
print("Task 1 started")
await asyncio.sleep(1)
print("Task 1 completed")
async def task2():
print("Task 2 started")
await asyncio.sleep(2)
print("Task 2 completed")
async def main():
task1 = asyncio.create_task(task1())
task2 = asyncio.create_task(task2())
await task1
await task2
asyncio.run(main())
In this example, both tasks are executed concurrently, but their completion times are different. This demonstrates how the event loop allows Python to manage multiple tasks without blocking any of them.
Handling Concurrency with asyncio
Concurrency in Python can be efficiently managed using asyncio
. While tasks can run concurrently, they don’t necessarily run in parallel. Instead, they yield control back to the event loop when waiting for I/O operations.
The following example shows how to handle multiple coroutines concurrently using asyncio.gather()
:
import asyncio
async def download_file(file_name):
print(f"Starting download: {file_name}")
await asyncio.sleep(3)
print(f"Download finished: {file_name}")
async def main():
files = ['file1.txt', 'file2.txt', 'file3.txt']
await asyncio.gather(*(download_file(file) for file in files))
asyncio.run(main())
Here, asyncio.gather()
allows us to run multiple downloads concurrently, making the code more efficient compared to running them sequentially.
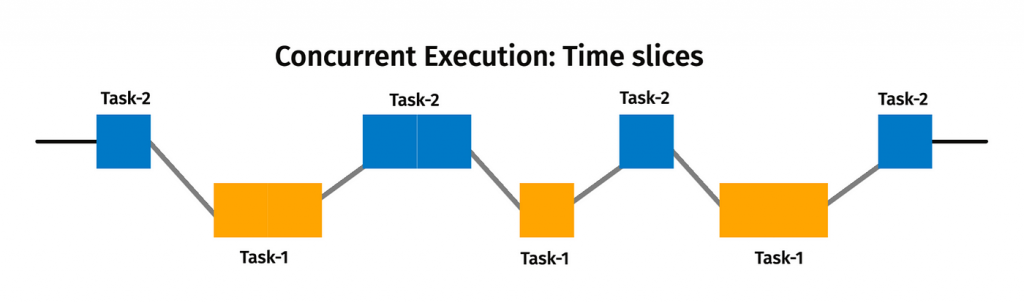
Best Practices for Asynchronous Programming
- Use
async
andawait
only for I/O-bound tasks, not CPU-bound tasks. - Keep your coroutines small and focused on one task.
- Avoid blocking the event loop with synchronous code.
- Use tools like aiohttp for asynchronous HTTP requests.
- Test your asynchronous code with tools like pytest-asyncio.
Conclusion
In this article, we explored the fundamentals of asynchronous programming in Python and learned how to use async
and await
to write more efficient, non-blocking code. We also covered the importance of event loops, tasks, and how to handle concurrency effectively using asyncio
. By mastering these techniques, you can create high-performance applications that handle I/O-bound tasks in a more efficient and scalable way.
Asynchronous programming is a powerful tool that every Python developer should have in their toolkit. If you follow the best practices and understand the concepts covered in this guide, you’ll be able to write more efficient and responsive code.
Leave a Reply